نوشتهها
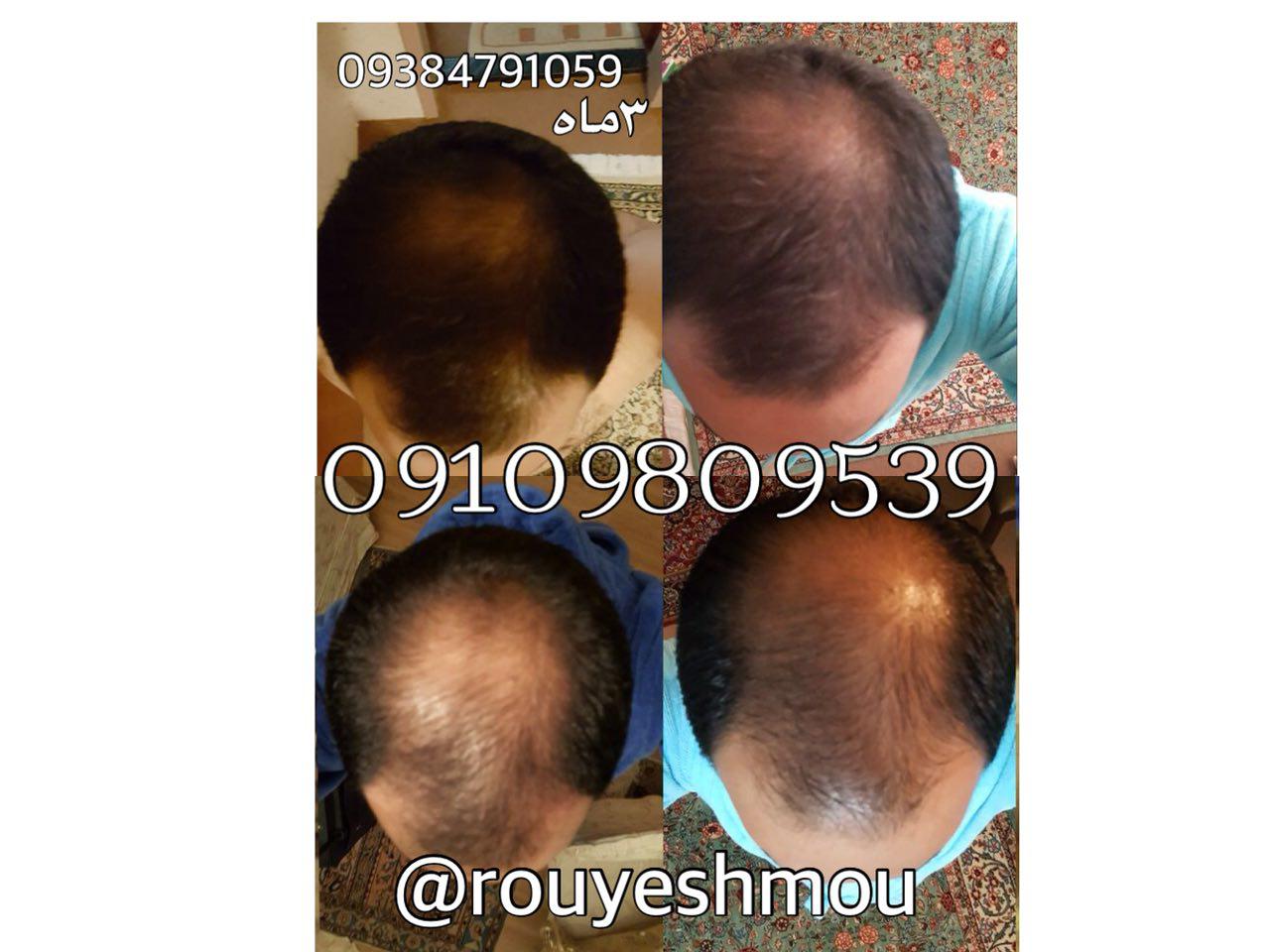
شرکت رویش سبز لاوین محلول رویش مو
شرکت رویش سبز لاوین محلول رویش مو
مشکل [bt_highlight]ریزش مو[/bt_high…
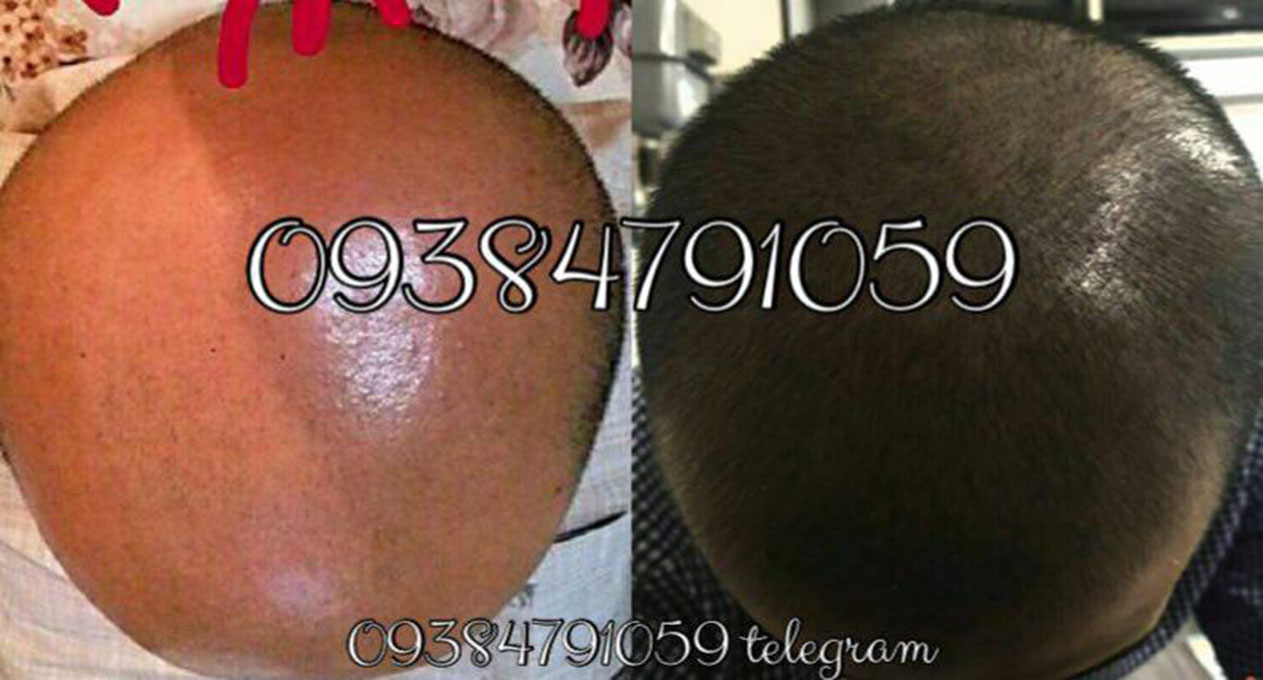
لیست نمایندگی های مجاز شرکت رویش سبز لاوین
لیست نمایندگی های مجاز شرکت رویش سبز لاوین
[bt_highlight]نمایند…
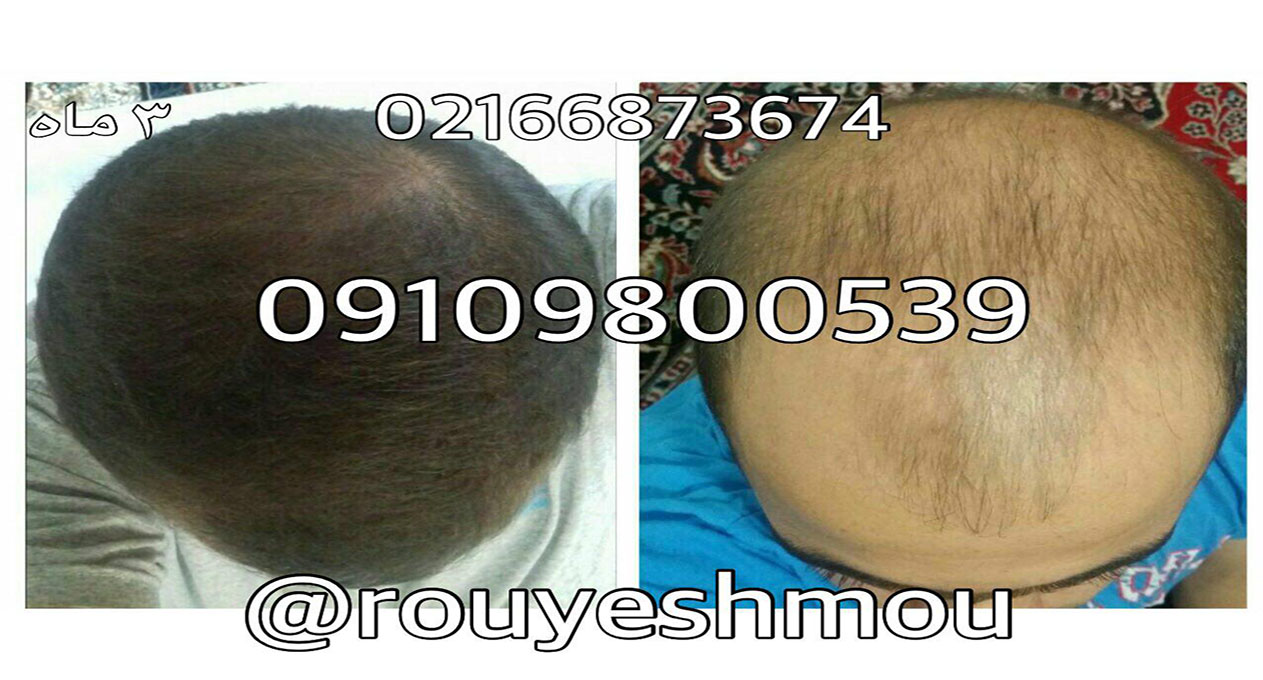
نمایندگی کرج شرکت رویش سبز لاوین
نمایندگی کرج شرکت رویش سبز لاوین
[bt_highlight]نمایندگی کرج شرکت رویش سبز لاوین[/bt_highli…
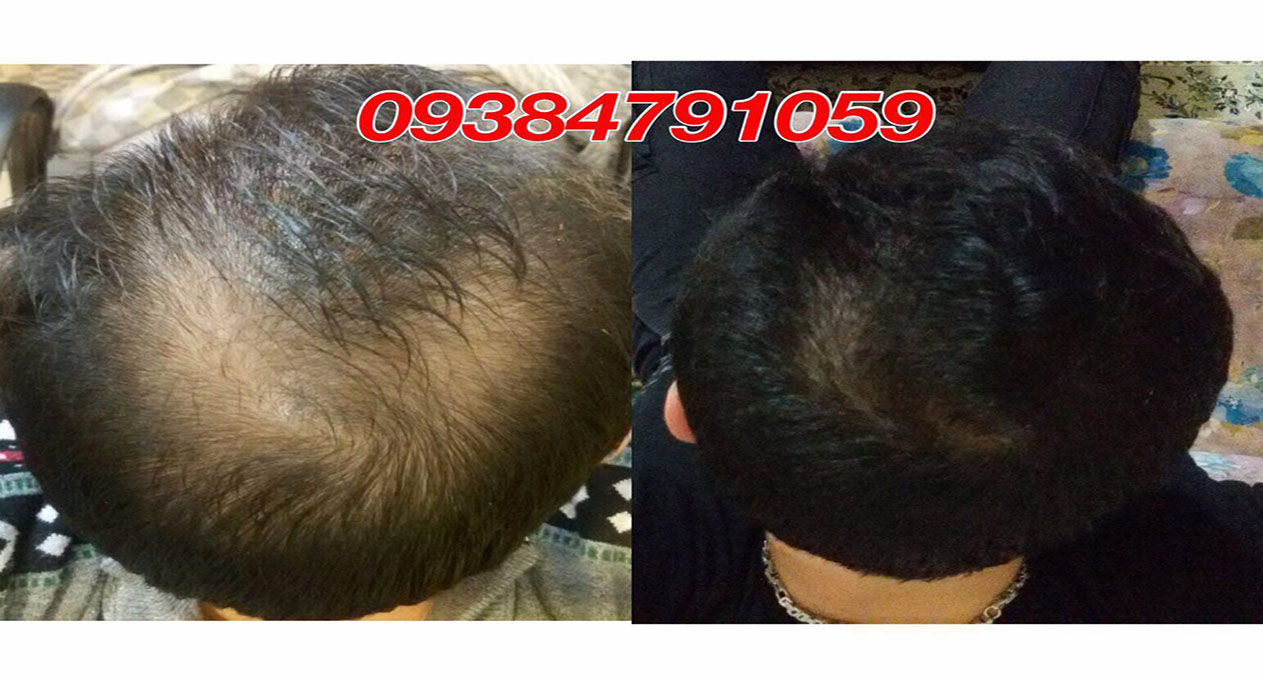
رشد مو شرکت رویش سبز لاوین
رشد مو شرکت رویش سبز لاوین
برای بسیاری از خانم ها رشد سر…
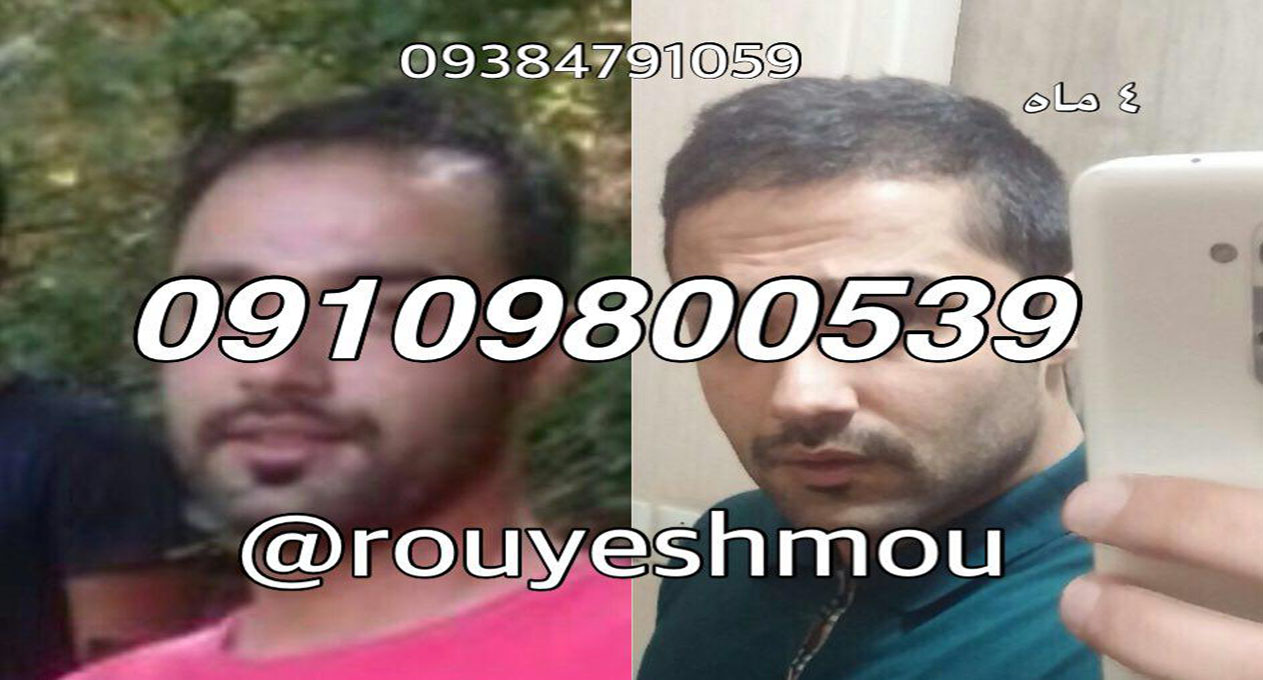
واقعيت هايي درمورد شرکت رویش سبز لاوین
واقعيت هايي درمورد شرکت رویش سبز لاوین
محصول عجيبي كه اين روزها تقريبا ٩٠٪ تهرا…
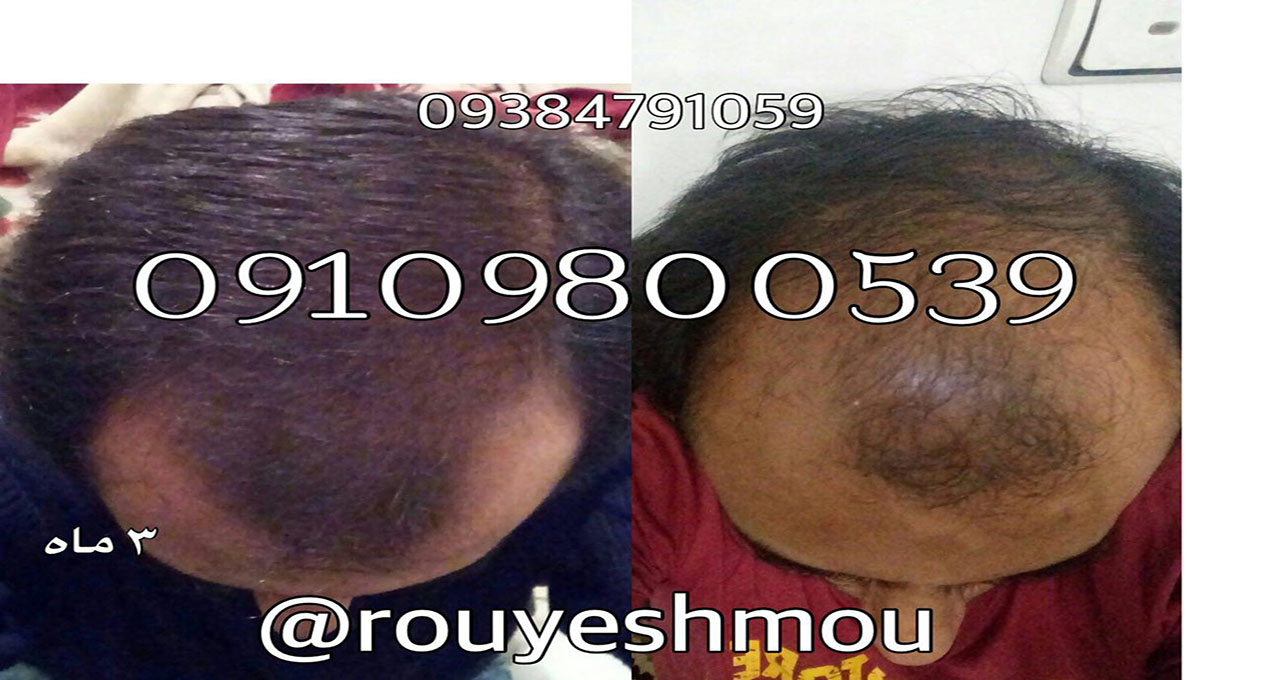
طرز استفاده از محلول شرکت رویش سبز لاوین
طرز استفاده از محلول شرکت رویش سبز لاوین
امروزه یکی از دغدغ…
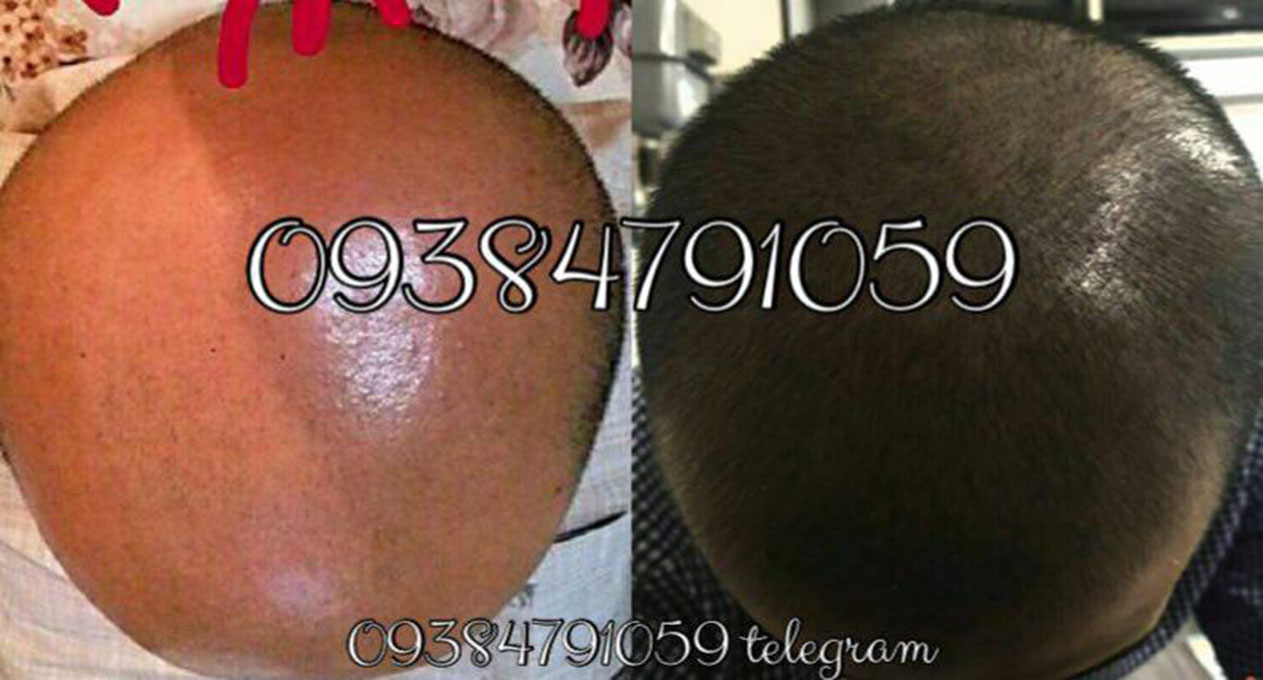
محلول شرکت رویش سبز لاوین
محلول شرکت رویش سبز لاوین
محلول شرکت رویش سبز لاوین علاوه…
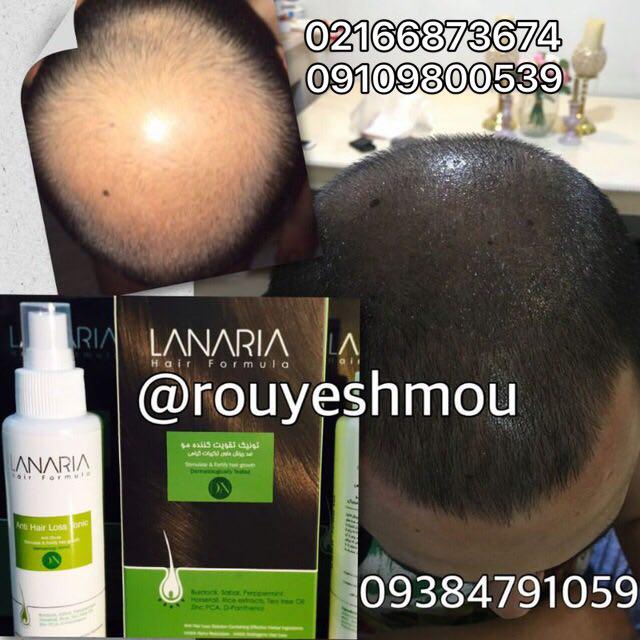
شرکت رویش سبز لاوین
[bt_highlight]شرکت رویش سبز لاوین[/bt_highlight]
در وحله اول با شنیدن…
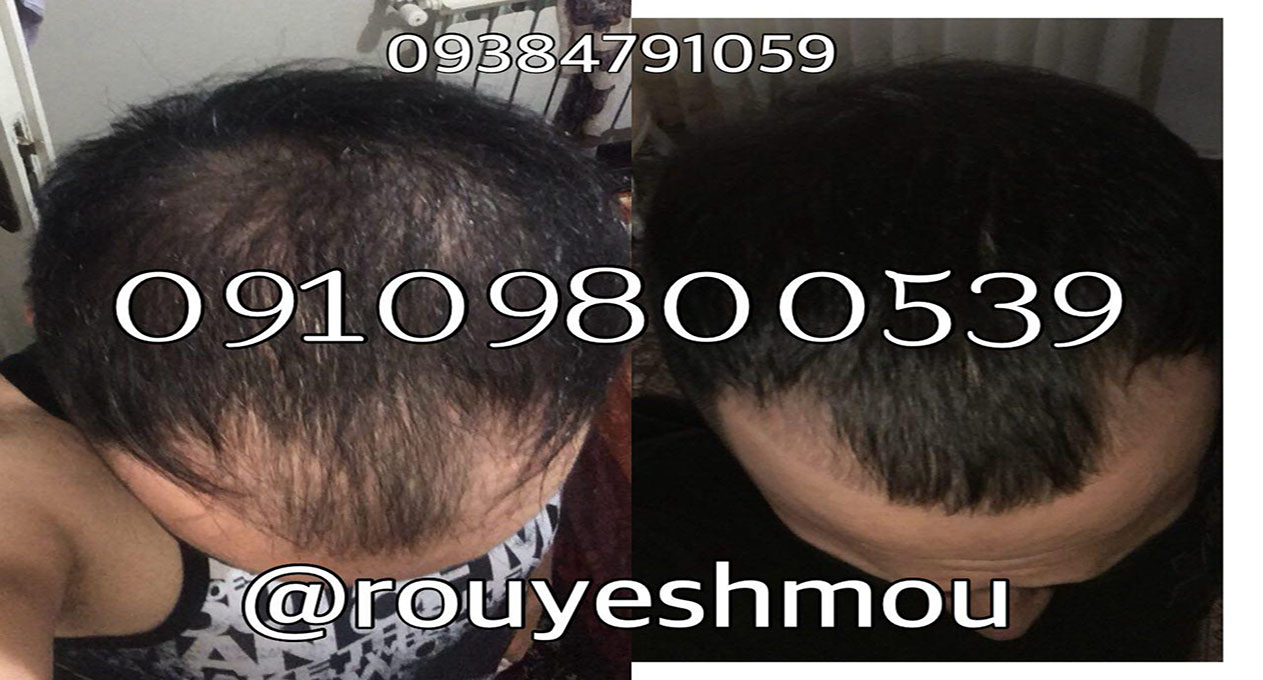
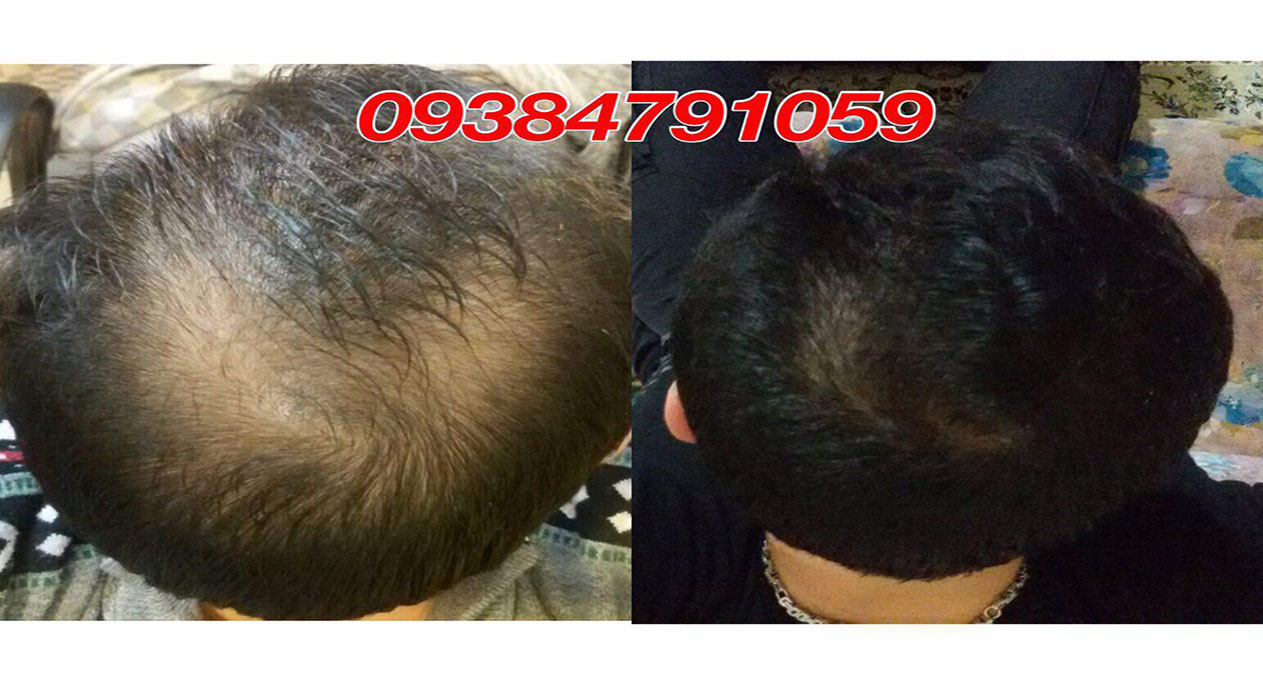
رشد موی شرکت رویش سبز لاوین
رشد موی شرکت رویش سبز لاوین
رشد موی شرکت رویش سبز لاوین
…
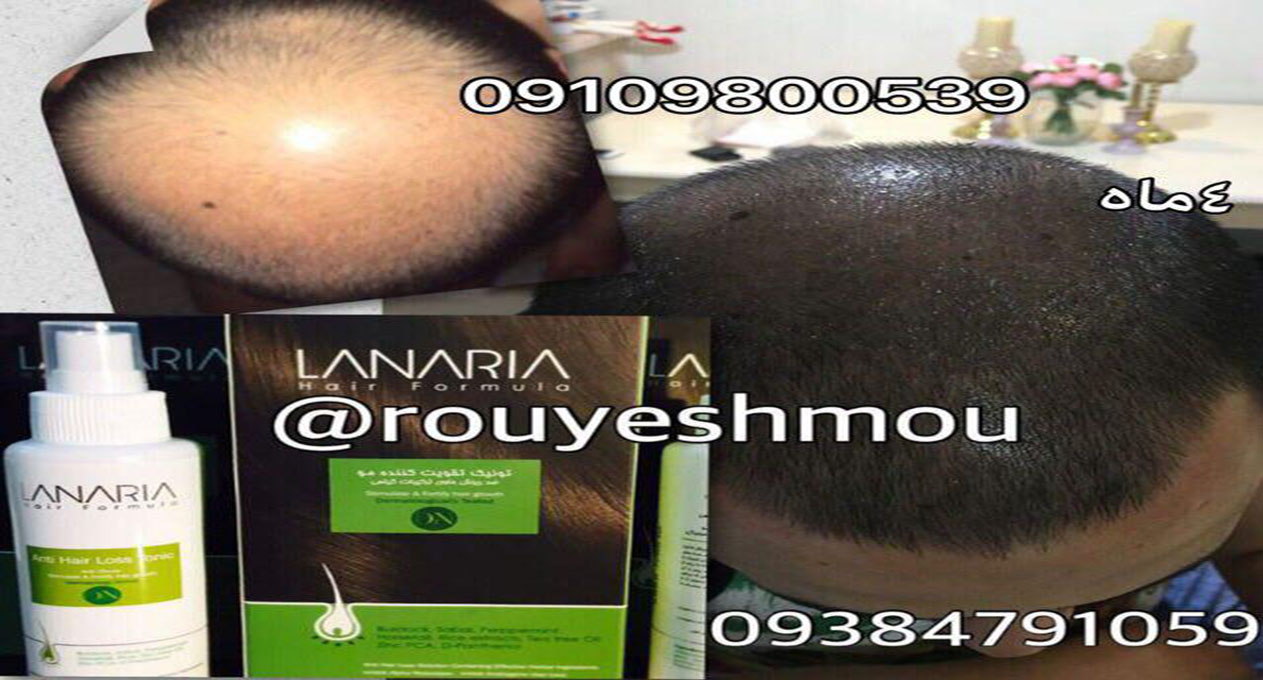
محلول شرکت رویش سبز لاوین
محلول شرکت رویش سبز لاوین
محلول شرکت رویش سبز لاوین
ریزش…
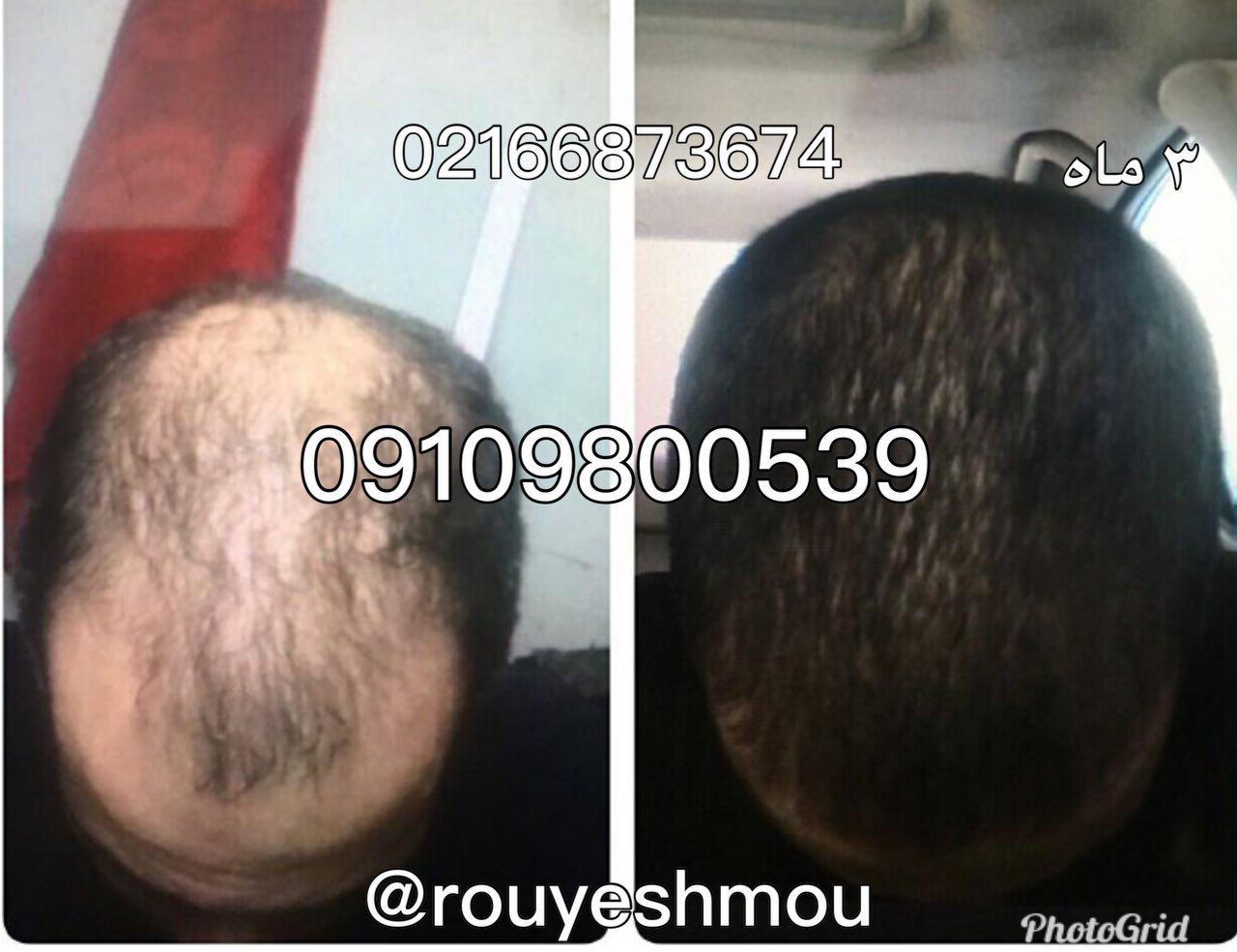
ماسک های تقویتی مو
ماسک های تقویتی مو
ماسک موی روغن نارگیل و رزماری:
دوقاش…